今天瓦特歐要來介紹,當各位朋友寫了一個Python code給其他人使用,但是對方
還是不知道怎麼使用,或者是需要一個介面,夾帶檔案路徑讓使用者好用,就來看
今天的文章,Python如何製作GUI,我們會使用JupyterNotebook開發環境來開發,那就開始今天的GUI製作吧~今天要介紹的是 grid
geometry manage,grid
顧明思義,就是要以網格的方式來討論布局視窗介面,相對於pack,是需要提供
較多的設定值,但是也能做出更精確的layout方式。
import tkinter as tk
from tkinter import * #pack is for tkinter
global root
root = Tk()
root.geometry('580x140')## Set window size#500
root.title("My first GUI")
root.mainloop()
GUI視窗:
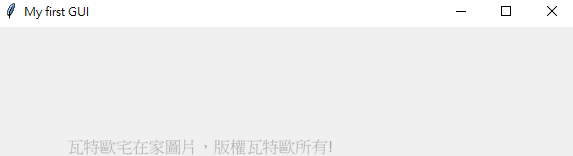
解說:
import tk 套件,創造一個叫做root的GUI主視窗,GUI視窗名稱My first GUI
學會開一個主視窗之後,要探討如何定義col和row的比例,這時候要請出
columnconfigure 和 rowconfigure設定,這兩個設定使用方式一模一樣
需要兩個參數: 1. col/row 2. weight
import tkinter as tk
root = tk.Tk()
root.geometry("600x400")
root.columnconfigure(0, weight=1)
root.columnconfigure(1, weight=2)
root.mainloop()
解說: root主視窗的,column 0 為基礎寬度,column 1 為column0的兩倍寬
import tkinter as tk
root = tk.Tk()
root.geometry("600x400")
root.columnconfigure((0, 2, 3), weight=1)
root.columnconfigure(1, weight=2)
root.mainloop()
解說:
root主視窗的,column 0 , 2, 3為基礎寬度,column 1 為其他column的兩
倍寬
PS:各位朋友去跑可能看不出來,有沒有兩倍寬~就讓瓦特歐繼續帶使用Grid帶
大家放元件,看看columnconfigure的效果,rowconfigure和columnconfigure
用法一樣,各位朋友可以自己試試。
import tkinter as tk
root = tk.Tk()
root.geometry("600x400")
root.columnconfigure(0, weight=1)
root.columnconfigure(1, weight=2)
rectangle_1 = tk.Label(root, text="Region 1", bg="magenta", fg="black")
rectangle_1.grid(column=0, ipadx=10, ipady=10)
rectangle_2 = tk.Label(root, text="Region 2", bg="cyan", fg="black")
rectangle_2.grid(column=1, ipadx=10, ipady=10)
root.mainloop()
解說:
創造label元件、顯示為Region1,背景色為magenta,字的顏色為black
放到colmun 0
創造label2元件、顯示為Region2,背景色為cyan,字的顏色為black
放到colmun 1
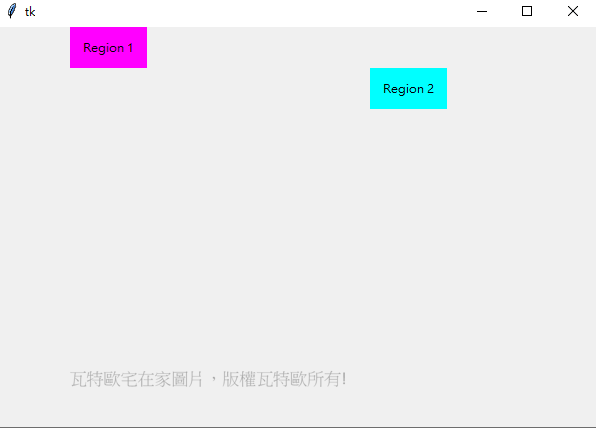
各位朋友們,看到圖片怎麼會跟原本說好的不一樣!!! Column 1應該要為Column
0的兩倍,請聽我娓娓道來,首先我們指定兩個label只有column並沒有指定row
放的位置,設定都是預設值。
import tkinter as tk
root = tk.Tk()
root.geometry("600x400")
root.columnconfigure(0, weight=1)
root.columnconfigure(1, weight=2)
rectangle_1 = tk.Label(root, text="Region 1", bg="magenta", fg="black")
rectangle_1.grid(column=0,row=0, ipadx=10, ipady=10)
rectangle_2 = tk.Label(root, text="Region 2", bg="cyan", fg="black")
rectangle_2.grid(column=1,row=0, ipadx=10, ipady=10)
root.mainloop()
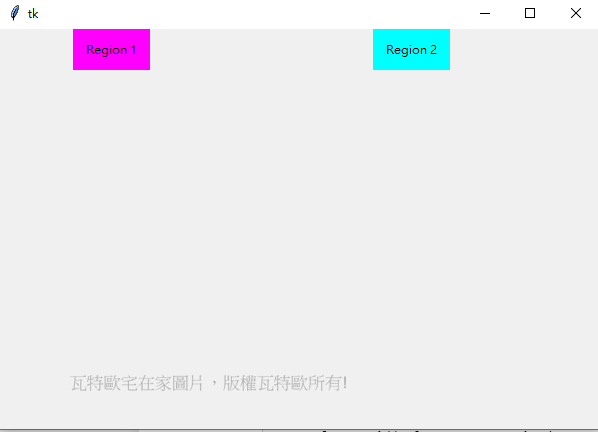
我們兩個label的row位置也補上了,瓦特歐!!!怎麼還是沒有兩倍寬?
原因是因為沒有指定元件的大小,但是如果layout都要指定元件大小,這樣是不是
太累人了!!!這時候就要請出另外一個好參數Sticky幫助我們填充元件大小!
import tkinter as tk
root = tk.Tk()
root.geometry("600x400")
root.columnconfigure(0, weight=1)
root.columnconfigure(1, weight=2)
rectangle_1 = tk.Label(root, text="Region 1", bg="magenta", fg="black")
rectangle_1.grid(column=0,row=0, ipadx=10, ipady=10, sticky="EW")
rectangle_2 = tk.Label(root, text="Region 2", bg="cyan", fg="black")
rectangle_2.grid(column=1,row=0, ipadx=10, ipady=10, sticky="EW")
root.mainloop()
解說:
sticky 可以輸入N ,S, E, W或是 混搭例如:EW,NS,NSEW,代表靠N(北方)
、S(南方)、E(東方)、W(西方),NS(北南延伸),EW(東西延伸),NSEW(全方位延
伸),我們
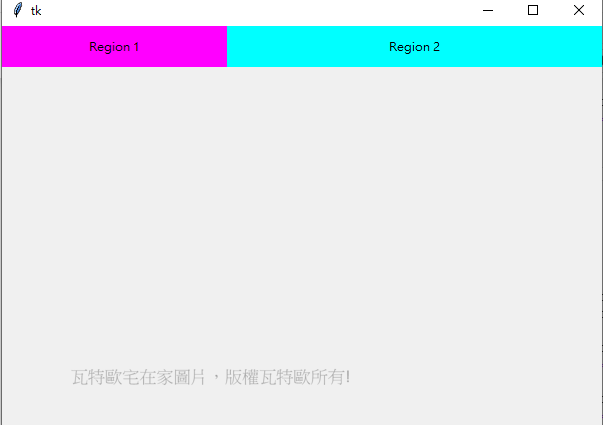
前面講解完,如何精準的擺放元件後,瓦特歐直接在這邊提供一個範例,給大家參
考參考!
完整layout範例code:
from tkinter import *
root = Tk()
root.title('My first GUI')
root.geometry('{}x{}'.format(460, 350))
# create all of the main containers
top_frame = Frame(root, bg='cyan', width=450, height=50, pady=3)
center = Frame(root, bg='gray2', width=50, height=40, padx=3, pady=3)
# layout all of the main containers
root.grid_rowconfigure(1, weight=2)
root.grid_columnconfigure(0, weight=1)
top_frame.grid(row=0, sticky="ew")
center.grid(row=1, sticky="nsew")
# create the widgets for the top frame
model_label = Label(top_frame, text='Model Dimensions')
width_label = Label(top_frame, text='Width:')
length_label = Label(top_frame, text='Length:')
entry_W = Entry(top_frame, background="pink")
entry_L = Entry(top_frame, background="orange")
# layout the widgets in the top frame
model_label.grid(row=0, columnspan=3)
width_label.grid(row=1, column=0)
length_label.grid(row=1, column=2)
entry_W.grid(row=1, column=1)
entry_L.grid(row=1, column=3)
# create the center widgets
center.grid_rowconfigure(0, weight=1)
center.grid_columnconfigure(1, weight=1)
ctr_left = Frame(center, bg='blue', width=100, height=190)
ctr_mid = Frame(center, bg='yellow', width=250, height=190, padx=3, pady=3)
ctr_right = Frame(center, bg='green', width=100, height=190, padx=3, pady=3)
ctr_left.grid(row=0, column=0, sticky="ns")
ctr_mid.grid(row=0, column=1, sticky="nsew")
ctr_right.grid(row=0, column=2, sticky="ns")
root.mainloop()
解說:
上述這範例code,提供一個Layout 大家方便好管理的觀念,分區寫主視窗、
各區域layout後,再開始放置元件,從最上層往下放元件,當介面做很大時、
才方便各位朋友找對應的位置去改Layout與元件位置。
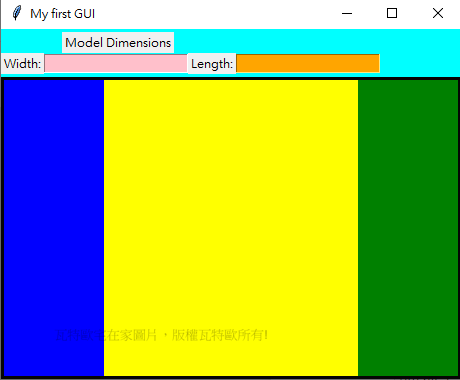
瓦特歐Python介紹系列:
[TIL#1] Python 自學 day1 Anaconda
[TIL#2] Python 自學 day2 變數
[TIL#3] Python 自學 day3 流程控制
[TIL#4] Python 自學 day4 製作執行檔
[TIL#5] Python 自學 day5 執行檔更換icon
[TIL#6] Python 自學 day6 PIL浮水印、圖片大小變更
[TIL#7] Python 自學 day7 大量圖片Resize 處理 懶人包
[TIL#8] Python 自學 day8 GUI製作- 使用Tkinter Grid管理器
[TIL#9] Python 自學 day9 GUI製作 放入圖片 grid 版本
[TIL#10] Python 自學 day10 創造圖片的拼貼
[TIL#11] Python 自學 day11 matplotlib 統計圖、長條圖、圓餅圖、散佈圖